How to Solve ReCaptcha v2 in Python using Selenium & CapMonster Cloud
If you want to write a script to automatically solve different types of captchas in Python, then first you will need to download the latest version of Python from the official website.
Next steps:
Create a folder in a convenient place, go to VS Code and add this folder to our new project (File – Open folder).
To add the Selenium dependency, go to the website https://www.selenium.dev/downloads/, select python, copy the line from above pip install selenium, open the terminal in our project (View – Terminal), paste this line there, press Enter and wait dependency installations.
Now you need to add the library from CapMonster Cloud, you can find out all the information about it here – https://pypi.org/project/capmonstercloudclient/. Copy the line pip install capmonstercloudclient and add it to the project via the terminal in the same way. You can check all installed dependencies using the pip list command.
Create a new file called “.env” and enter the following values there:
API_KEY="YOUR_API_KEY"
WEBSITE_KEY="6Le-wvkSAAAAAPBMRTvw0Q4Muexq9bi0DJwx_mJ-"
WEBSITE_URL="https://google.com/recaptcha/api2/demo"
For example, we take the site google.com/recaptcha/api2/demo, it has a captcha that we are going to solve using CapMonster Cloud, there is a sitekey identifier for it. API_KEY is your API key from CapMonster Cloud, enter it in the appropriate line and save it in the “.env” file.
Create a “main.py” file and import all the necessary modules, dependencies and environment variable settings from the “.env” file (dotenv should be installed in your dependencies):
import asyncio
from selenium import webdriver
from selenium.webdriver.common.by import By
from capmonstercloudclient import CapMonsterClient, ClientOptions
from capmonstercloudclient.requests import RecaptchaV2ProxylessRequest
from dotenv import load_dotenv
import os
load_dotenv()
API_KEY = os.getenv("API_KEY")
WEBSITE_URL = os.getenv("WEBSITE_URL")
WEBSITE_KEY = os.getenv("WEBSITE_KEY")
- Next, we write the code to launch the browser (in our example we use webdriver.Chrome, you may have a different driver, don’t forget about it!), create a task to solve the captcha and insert the result on the target site:
client_options = ClientOptions(api_key=API_KEY)
cap_monster_client = CapMonsterClient(options=client_options)
async def solve_captcha():
# Creating a reCAPTCHA solution request type V2 Proxyless
recaptcha_request = RecaptchaV2ProxylessRequest(websiteUrl=WEBSITE_URL, websiteKey=WEBSITE_KEY)
result = await cap_monster_client.solve_captcha(recaptcha_request)
return result['gRecaptchaResponse']
async def main():
try:
# Running Chrome Browser with Selenium
browser = webdriver.Chrome()
# Opening a website
browser.get(WEBSITE_URL)
print('Page open, waiting for reCAPTCHA to load...')
# Wait a while for the CAPTCHA to load (you can configure the duration)
await asyncio.sleep(5)
# reCAPTCHA solution using CapMonster
responses = await solve_captcha()
print('CapMonster response:', responses)
# Inserting a solved reCAPTCHA into a page
browser.execute_script(f'document.getElementById("g-recaptcha-response").innerHTML = "{responses}";')
print('The result is inserted into the page!')
# Performing additional actions if necessary (for example, submitting a form)
browser.find_element(By.ID, 'recaptcha-demo-submit').click()
print('Form submitted!')
# Wait a while to observe changes
await asyncio.sleep(10)
except Exception as e:
print('Error:', e)
finally:
# Closing the browser
browser.quit()
# Running an asynchronous event loop
asyncio.run(main())
Full code:
import asyncio
from selenium import webdriver
from selenium.webdriver.common.by import By
from capmonstercloudclient import CapMonsterClient, ClientOptions
from capmonstercloudclient.requests import RecaptchaV2ProxylessRequest
from dotenv import load_dotenv
import os
# Loading environment variables from an .env file
load_dotenv()
API_KEY = os.getenv("API_KEY")
WEBSITE_URL = os.getenv("WEBSITE_URL")
WEBSITE_KEY = os.getenv("WEBSITE_KEY")
# Setting up the CapMonster client
client_options = ClientOptions(api_key=API_KEY)
cap_monster_client = CapMonsterClient(options=client_options)
async def solve_captcha():
# Creating a reCAPTCHA solution request type V2 Proxyless
recaptcha_request = RecaptchaV2ProxylessRequest(websiteUrl=WEBSITE_URL, websiteKey=WEBSITE_KEY)
result = await cap_monster_client.solve_captcha(recaptcha_request)
return result['gRecaptchaResponse']
async def main():
try:
# Running Chrome Browser with Selenium
browser = webdriver.Chrome()
# Opening a website
browser.get(WEBSITE_URL)
print('Page open, waiting for reCAPTCHA to load...')
# Wait a while for the CAPTCHA to load (you can configure the duration)
await asyncio.sleep(5)
# reCAPTCHA solution using CapMonster
responses = await solve_captcha()
print('CapMonster response:', responses)
# Inserting a solved reCAPTCHA into a page
browser.execute_script(f'document.getElementById("g-recaptcha-response").innerHTML = "{responses}";')
print('The result is inserted into the page!')
# Performing additional actions if necessary (for example, submitting a form)
browser.find_element(By.ID, 'recaptcha-demo-submit').click()
print('Form submitted!')
# Wait a while to observe changes
await asyncio.sleep(10)
except Exception as e:
print('Error:', e)
finally:
# Closing the browser
browser.quit()
# Running an asynchronous event loop
asyncio.run(main())
Code Explanation:
load_dotenv() is used to load environment variables from the .env file.
os.getenv("API_KEY"), os.getenv("WEBSITE_URL"), and os.getenv("WEBSITE_KEY") are used to obtain the values of the corresponding environment variables from the .env file.
ClientOptions(api_key=API_KEY) creates a ClientOptions object with the given API key for CapMonster.
CapMonsterClient(options=client_options) creates a CapMonster client with the given options.
async def solve_captcha(): this function creates a CAPTCHA solution request of reCAPTCHA V2 Proxyless type using CapMonster.
Returns a gRecaptchaResponse value that represents the CAPTCHA solution.
async def main(): main asynchronous function that executes the main script.
Launches Chrome browser using Selenium, opens website, waits for reCAPTCHA to load.
Calls solve_captcha() to solve a CAPTCHA using CapMonster.
Inserts a solved CAPTCHA into a page, performs additional actions (such as submitting a form), and then waits for changes.
Catches and displays an error if something goes wrong.
Closes the browser after completing all the actions.
asyncio.run(main()) runs the asynchronous function main() using asyncio.run(), which allows you to run script-style asynchronous code.
Let's run our script. If any errors appear, use Run and Debug. If everything is correct, you should get this result:
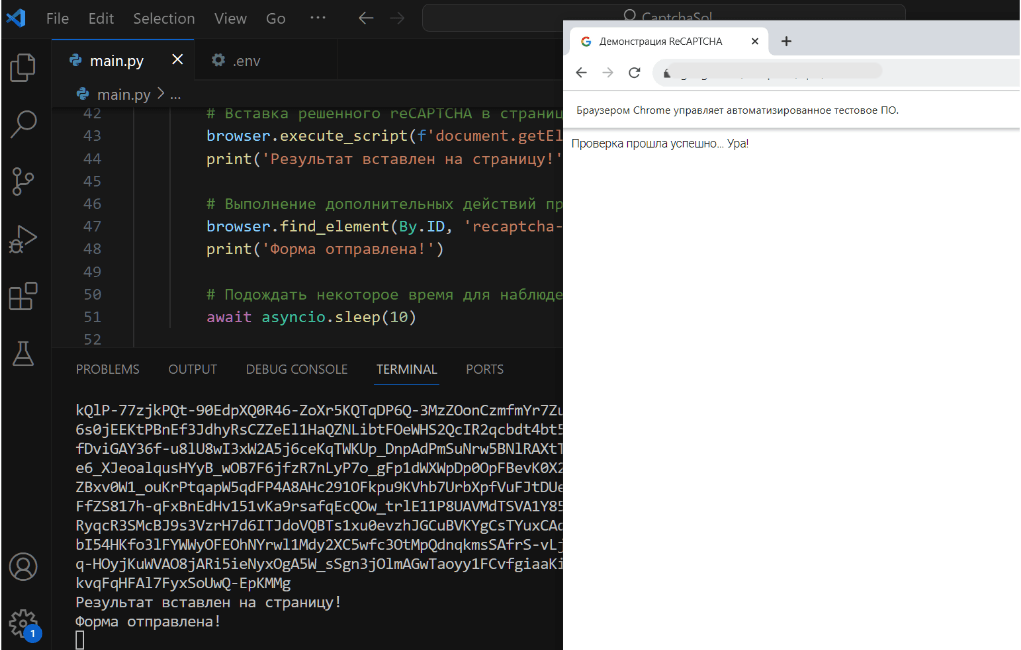
Note: We'd like to remind you that the product is used to automate testing on your own websites and on websites to which you have legal access.