How to Solve ReCaptcha v2 in JavaScript Using Selenium&CapMonster Cloud
We will run the code via Node.js, so download it in advance and install it on your computer.
- Next, create a folder for a new project, name it, for example, BypassRecaptchav2Js, launch VS Code and open the created folder.
- Create a “.env” file and add all the needed parameters there:
CAPMONSTER_API_KEY=YOUR_API_KEY
WEBSITE_KEY=6Le-wvkSAAAAAPBMRTvw0Q4Muexq9bi0DJwx_mJ-
WEBSITE_URL=https://www.google.com/recaptcha/api2/demo
- Load package.json with the command in the terminal: npm init, we need this file to be able to see a list of all installed modules and dependencies. Open a terminal and install the necessary packages with the command “npm i @zennolab_com/capmonstercloud-client dotenv selenium selenium-webdriver”.
- Create a file “main.js” and start writing code. First, let's import all the components and load data:
require('dotenv').config();
const { Builder, By, until } = require('selenium-webdriver');
const chrome = require('selenium-webdriver/chrome');
const { CapMonsterCloudClientFactory, ClientOptions, RecaptchaV2ProxylessRequest } = require('@zennolab_com/capmonstercloud-client');
const CAPMONSTER_API_KEY = process.env.CAPMONSTER_API_KEY;
const WEBSITE_KEY = process.env.WEBSITE_KEY;
const WEBSITE_URL = process.env.WEBSITE_URL;
- Now we need to launch the browser, find the captcha element and create a solving request in CapMonster Cloud:
async function solveRecaptcha() {
const options = new chrome.Options();
const driver = await new Builder().forBrowser('chrome').setChromeOptions(options).build();
try {
await driver.get(WEBSITE_URL);
console.log('Page open, reCAPTCHA solving...');
- Now let’s send a request with the created task to the server to solve reCAPTCHA:
// Initializing the CapMonster client
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));
// Creating a request for a reCAPTCHA solving V2 Proxyless type
const recaptchaV2ProxylessRequest = new RecaptchaV2ProxylessRequest({
websiteURL: WEBSITE_URL,
websiteKey: WEBSITE_KEY,
});
// reCAPTCHA solving using CapMonster
const solution = await cmcClient.Solve(recaptchaV2ProxylessRequest);
console.log('Solution from CapMonster:', solution);
// Retrieving a token from a solution object
const token = solution.solution.gRecaptchaResponse;
- We will implement the solution on the page and create a subsequent form submission after successfully solving the captcha:
// Inserting the result into a form
await driver.executeScript((result) => {
document.getElementById('g-recaptcha-response').value = result;
console.log('Token in the console:', result); // Outputting the token to the console
}, token);
console.log('The token is inserted into the form!');
await driver.findElement(By.id('recaptcha-demo-submit')).click();
console.log('Form submitted!'); // Optional step
// Pause for 10 seconds
await new Promise(resolve => setTimeout(resolve, 10000));
} catch (error) {
console.error('Error:', error);
} finally {
await driver.quit();
}
}
solveRecaptcha();
Full code:
require('dotenv').config();
const { Builder, By, until } = require('selenium-webdriver');
const chrome = require('selenium-webdriver/chrome');
const { CapMonsterCloudClientFactory, ClientOptions, RecaptchaV2ProxylessRequest } = require('@zennolab_com/capmonstercloud-client');
const CAPMONSTER_API_KEY = process.env.CAPMONSTER_API_KEY;
const WEBSITE_KEY = process.env.WEBSITE_KEY;
const WEBSITE_URL = process.env.WEBSITE_URL;
async function solveRecaptcha() {
const options = new chrome.Options();
const driver = await new Builder().forBrowser('chrome').setChromeOptions(options).build();
try {
await driver.get(WEBSITE_URL);
console.log('Page open, reCAPTCHA solving...');
// Initializing the CapMonster client
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));
// Creating a request for a reCAPTCHA solving V2 Proxyless type
const recaptchaV2ProxylessRequest = new RecaptchaV2ProxylessRequest({
websiteURL: WEBSITE_URL,
websiteKey: WEBSITE_KEY,
});
// reCAPTCHA solution using CapMonster
const solution = await cmcClient.Solve(recaptchaV2ProxylessRequest);
console.log('Solution from CapMonster:', solution);
// Retrieving a token from a solution object
const token = solution.solution.gRecaptchaResponse;
// Inserting the result into a form
await driver.executeScript((result) => {
document.getElementById('g-recaptcha-response').value = result;
console.log('Token in the console:', result); // Outputting the token to the console
}, token);
console.log('The token is inserted into the form!');
await driver.findElement(By.id('recaptcha-demo-submit')).click();
console.log('Form submitted!'); // Optional step
// Pause for 10 seconds
await new Promise(resolve => setTimeout(resolve, 10000));
} catch (error) {
console.error('Error:', error);
} finally {
await driver.quit();
}
}
solveRecaptcha();
Code explanation:
Importing libraries:
dotenv is used to load environment variables from the .env file.
selenium-webdriver is used to automate the browser using WebDriver.
selenium-webdriver/chrome provides options to customize the Chrome browser.
@zennolab_com/capmonstercloud-client provides tools for working with CapMonster Cloud.
Loading Environment Variables: dotenv is used to load environment variables from the .env file such as CAPMONSTER_API_KEY, WEBSITE_KEY and WEBSITE_URL.
solveRecaptcha function: creates an instance of the Chrome driver using Selenium WebDriver.
Initializes the CapMonster client using the loaded CAPMONSTER_API_KEY.
Goes to a website with reCAPTCHA.
Creates a request for a reCAPTCHA V2 Proxyless type solution.
Solves reCAPTCHA using CapMonster and gets a solution.
Inserts the resulting token (solution) into a form on a web page using JavaScript.
Optionally submits the form by clicking on the button with id 'recaptcha-demo-submit'.
Waits 10 seconds after solving.
Terminates the driver.
try-catch-finally block:
The try block contains the main automation execution code.
The catch block handles any exceptions and prints an error message.
The finally block ensures that the driver is closed even if an error occurs.
Calling solveRecaptcha() function: runs the solveRecaptcha() function to solve reCAPTCHA when executing the script.
Let's run our code with the command in the terminal: node main.js and see what happens:
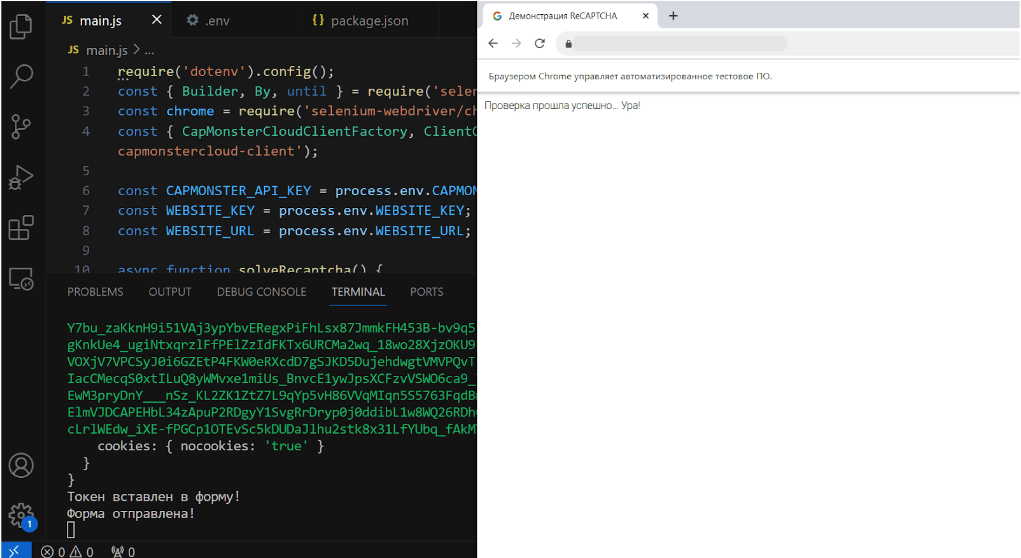
Note: We'd like to remind you that the product is used to automate testing on your own websites and on websites to which you have legal access.