Solving Complex CAPTCHAs with Puppeteer and Playwright Using CapMonster Cloud
Puppeteer and Playwright are libraries for automating actions in the browser. With their help, you can control the browser and perform any work, such as opening and navigating pages, filling out forms, clicking buttons, extracting data and other tasks. You can also automate solving captchas on websites if you add the use of the CapMonster Cloud service. Next, we will look at their integration and provide ready-made code examples that can be used to automatically bypass captchas.
Puppeteer and Playwright are not much different from each other. Puppeteer was created by the Google team. It is a Node.js library running on JavaScript. It has its own built-in browser based on the Chromium engine; you don’t need to manually install a web driver, as necessary, for example, in Selenium. Playwright is developed by Microsoft, it is more flexible and slightly expands the capabilities of Puppeteer, allowing you to work in several languages, including JavaScript, TypeScript, Python, C# and others. It, like Puppeteer, has several built-in browsers, so you will be able to test it in Chromium, Firefox and WebKit (Safari). Let's look at using Puppeteer and Playwright in conjunction with CapMonster Cloud.
Puppeteer
We’ll be using VS Code, so we will focus on this environment. All code examples will run without using a proxy. If you need to specify a proxy, just additionally specify their parameters and the current user-agent when creating a task, for example:
"clientKey":"YOUR_API_KEY",
"task": {
"type":"RecaptchaV2Task",
"websiteURL":"https://lessons.zennolab.com/captchas/recaptcha/v2_simple.php?level=high",
"websiteKey":"EXAMPLE_SITE_KEY",
"proxyType":"http",
"proxyAddress":"8.8.8.8",
"proxyPort":8080,
"proxyLogin":"proxyLoginHere",
"proxyPassword":"proxyPasswordHere",
"userAgent":"Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/126.0.0.0 Safari/537.36"
So, to install Puppeteer, you need to follow these steps:
- Installing Node.js. Puppeteer requires Node.js, so make sure you have the latest version installed.
- Creating a project. Create a new directory for your project if you don't already have one.
- Initializing Node.js. Open a terminal in VS Code (press Ctrl + ~ to open the integrated terminal), go to your directory and run npm init, then follow the instructions in the terminal. This command will initiate a new Node.js project and create a package.json file. All installed dependencies will appear in this file.
- Installing Puppeteer. In the VS Code terminal, run npm install puppeteer to download Puppeteer and its dependencies into your project.
Now you need to install a library convenient for you to send and receive HTTP requests. CapMonster Cloud has its own library for this, and we will use it in our examples. Install it with the command npm i @zennolab_com/capmonstercloud-client.
ReCaptcha v.2
You need to find out the sitekey of the captcha and other data necessary to successfully send the request, receive an answer and insert the token into the captcha element. All this can be found in the code in the developer tools. Also be sure to check out the official CapMonster Cloud documentation.
- Let's create a new project in JavaScript and import all the necessary dependencies:
import { launch } from 'puppeteer';
import { CapMonsterCloudClientFactory, ClientOptions, RecaptchaV2ProxylessRequest } from '@zennolab_com/capmonstercloud-client';
- Next, set the values (by the way, for convenience and to avoid data leakage, it is better to create a separate .env file in the same directory and write all this data there, using them in code using the dotenv library installed and imported into the project):
const CAPMONSTER_API_KEY = 'YOUR_API_KEY';
const WEBSITE_KEY = 'EXAMPLE_SITE_KEY';
const WEBSITE_URL = 'https://lessons.zennolab.com/captchas/recaptcha/v2_simple.php?level=high';
- Let's create a task, send it to the CapMonster Cloud server, get a solution and insert a token into the captcha form:
async function solveRecaptcha() {
const browser = await launch({ headless: false });
const page = await browser.newPage();
// Initializing the CapMonster client
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));
try {
await page.goto(WEBSITE_URL);
console.log('Page open, reCAPTCHA solving...');
// Creating a request for a reCAPTCHA solving type V2 Proxyless
const recaptchaV2ProxylessRequest = new RecaptchaV2ProxylessRequest({
websiteURL: WEBSITE_URL,
websiteKey: WEBSITE_KEY,
});
// reCAPTCHA solving using CapMonster
const solution = await cmcClient.Solve(recaptchaV2ProxylessRequest);
console.log('Solution from CapMonster:', solution);
// Retrieving a token from a solution object
const token = solution.solution.gRecaptchaResponse;
// Inserting the result into a form
await page.evaluate((result) => {
document.getElementById('g-recaptcha-response').value = result;
console.log('Token in the console:', result); // Output the token to the console
}, token);
console.log('The token is inserted into the form!');
await page.click('#recaptcha-demo-submit');
console.log('Form submitted!'); // Optional step
await new Promise(resolve => setTimeout(resolve, 10000));
} catch (error) {
console.error('Error:', error);
} finally {
await browser.close();
}
}
solveRecaptcha();
Code Explanation:
async function solveRecaptcha() {...}: Declaring an asynchronous solveRecaptcha function that will contain all the code.
const browser = await launch({ headless: false });: Launching a Browser with Puppeteer. headless: false option means that the browser will be launched with a GUI (not in the background)
const page = await browser.newPage();: Creating a new tab in the browser.
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));: Initializing the CapMonster client using the provided API key.
await page.goto(WEBSITE_URL);: Going to the specified website.
const recaptchaV2ProxylessRequest = new RecaptchaV2ProxylessRequest({ ... });: Creating a request object for reCAPTCHA solving type V2 Proxyless. Here you set parameters such as website URL and reCAPTCHA key.
const solution = await cmcClient.Solve(recaptchaV2ProxylessRequest);: Sending a request to CapMonster to solve reCAPTCHA. The result is saved in the solution object.
const token = solution.solution.gRecaptchaResponse;: Retrieving a token (reCAPTCHA response) from a CapMonster solution object.
await page.evaluate((result) => {...}, token);: Inserting a token into a form on a web page using Puppeteer's evaluate method.
await page.click('#recaptcha-demo-submit');: Clicking on a button with the specified selector (in this case, a button with id recaptcha-demo-submit).
await new Promise(resolve => setTimeout(resolve, 10000));: Wait 10 seconds (in this case, pause) using Promise.
} catch (error) { console.error('Error:', error); } finally { await browser.close(); }}: Error handling (if any) and closing the browser in the finally block.
solveRecaptcha();: Calling the solveRecaptcha function to complete the entire process.
Full code:
import { launch } from 'puppeteer';
import { CapMonsterCloudClientFactory, ClientOptions, RecaptchaV2ProxylessRequest } from '@zennolab_com/capmonstercloud-client';
const CAPMONSTER_API_KEY = 'YOUR_API_KEY';
const WEBSITE_KEY = 'EXAMPLE_SITE_KEY';
const WEBSITE_URL = 'https://lessons.zennolab.com/captchas/recaptcha/v2_simple.php?level=high';
async function solveRecaptcha() {
const browser = await launch({ headless: false });
const page = await browser.newPage();
// Initializing the CapMonster client
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));
try {
await page.goto(WEBSITE_URL);
console.log('Page is open, reCAPTCHA solving...');
// Creating a request for a reCAPTCHA solving type V2 Proxyless
const recaptchaV2ProxylessRequest = new RecaptchaV2ProxylessRequest({
websiteURL: WEBSITE_URL,
websiteKey: WEBSITE_KEY,
});
// reCAPTCHA solving using CapMonster
const solution = await cmcClient.Solve(recaptchaV2ProxylessRequest);
console.log('Solution from CapMonster:', solution);
// Retrieving a token from a solution object
const token = solution.solution.gRecaptchaResponse;
// Inserting the result into a form
await page.evaluate((result) => {
document.getElementById('g-recaptcha-response').value = result;
console.log('Token is in the console:', result); // Output the token to the console
}, token);
console.log('The token is inserted into the form!');
await page.click('#recaptcha-demo-submit');
console.log('Form is submitted!'); // Optional step
await new Promise(resolve => setTimeout(resolve, 10000));
} catch (error) {
console.error('Error:', error);
} finally {
await browser.close();
}
}
solveRecaptcha();
- Run the code through the command in the terminal: node your_project_name.js or via the debug console. If successful, a confirmed page will open:
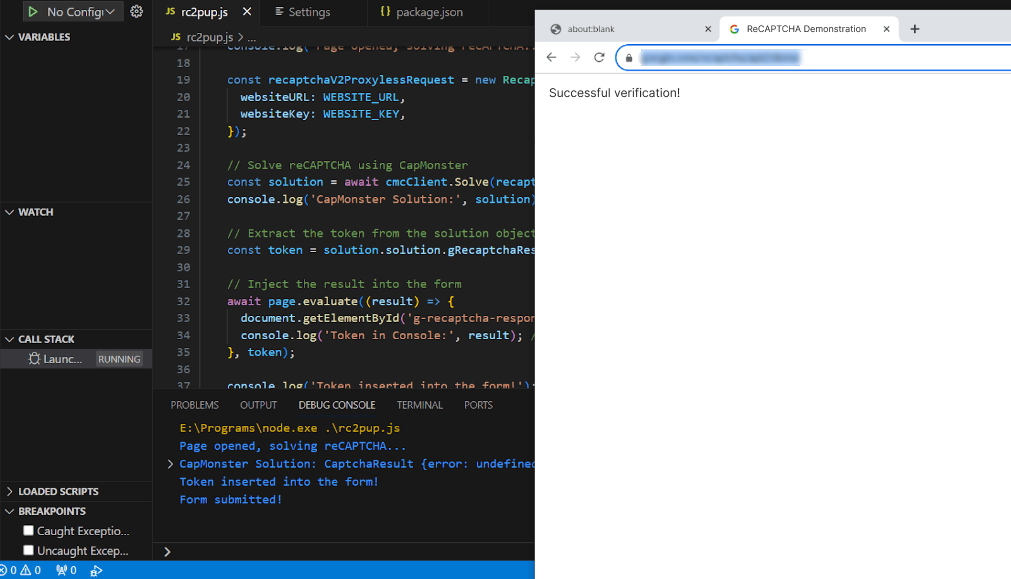
ReCaptcha v.3
- Import all the necessary dependencies:
import { launch } from 'puppeteer';
import { CapMonsterCloudClientFactory, ClientOptions, RecaptchaV3ProxylessRequest } from '@zennolab_com/capmonstercloud-client';
- Set the values:
const CAPMONSTER_API_KEY = 'YOUR_API_KEY';
const WEBSITE_KEY = 'EXAMPLE_SITE_KEY';
const WEBSITE_URL = 'https://lessons.zennolab.com/captchas/recaptcha/v3.php?level=beta';
- Let's create a task, send it to the CapMonster Cloud server, get a solution and insert a token into the captcha form:
async function solveRecaptcha() {
const browser = await launch({ headless: false });
const page = await browser.newPage();
console.log('Browser is initialized!');
// Initializing the CapMonster client
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));
try {
await page.goto(WEBSITE_URL, {
waitUntil: 'domcontentloaded'
});
console.log('Browser page is switched to captcha page!');
const recaptchaV3ProxylessRequest = new RecaptchaV3ProxylessRequest({
websiteURL: WEBSITE_URL,
websiteKey: WEBSITE_KEY,
minScore: 0.7,
pageAction: "verify",
});
// Receiving a captcha solution from CapMonster
const solution = await cmcClient.Solve(recaptchaV3ProxylessRequest);
console.log('CapMonster Solution:', solution);
// Installing a custom userAgent (optional)
await page.setUserAgent('Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/126.0.0.0 Safari/537.36'); // You can replace it with your own
await page.evaluate((result) => {
document.querySelector('#g-recaptcha-response-100000').style.display = 'block';
document.querySelector('#g-recaptcha-response-100000').style.visibility = 'visible';
document.querySelector('#g-recaptcha-response-100000').value = result;
}, solution);
console.log('Result injected into the page!');
await Promise.all([
page.waitForNavigation(),
page.click('#v3_submit')
]);
console.log('Button "Check" clicked!');
await new Promise(resolve => setTimeout(resolve, 10000));
} catch (e) {
console.error('An error occurred:', e);
} finally {
await browser.close();
}
}
solveRecaptcha();
Explanation of this part of the code:
async function solveRecaptcha() {...}: Declaring an asynchronous solveRecaptcha function that contains all the code.
const browser = await launch({ headless: false });: Launching a browser using Puppeteer. The headless: false option means that the browser will be launched with a GUI (not in the background).
const page = await browser.newPage();: Creating a new browser tab.
console.log('Browser is initialized!');: Output a message to the console that the browser is initialized.
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));: Initializing the CapMonster client using the provided API key.
await page.goto(WEBSITE_URL);: Going to the specified website.
const recaptchaV3ProxylessRequest = new RecaptchaV3ProxylessRequest({ ... });: Creating a request object for reCAPTCHA solving type V3 Proxyless. Here are set parameters such as website URL and reCAPTCHA key.
const solution = await cmcClient.Solve(recaptchaV3ProxylessRequest);: Submitting a request to CapMonster to solve reCAPTCHA. The result is saved in the solution object.
await page.evaluate((result) => {...}, solution);: Inserting a reCAPTCHA solution into a form on a web page using Puppeteer's evaluate method.
await Promise.all([...]);: Waiting for both navigation to complete and a button with the specified selector to be clicked.
console.log('Result injected into the page!');: Displaying a message to the console that the result has been inserted into the page.
await new Promise(resolve => setTimeout(resolve, 10000));: Wait 10 seconds (pause) using Promise.
} catch (e) { console.error('An error occurred:', e); } finally { await browser.close(); }}: Handling errors (if any occur) and closing the browser in a finally block.
solveRecaptcha();: Calling the solveRecaptcha function to complete the entire process.
Full code:
import { launch } from 'puppeteer';
import { CapMonsterCloudClientFactory, ClientOptions, RecaptchaV3ProxylessRequest } from '@zennolab_com/capmonstercloud-client';
const CAPMONSTER_API_KEY = 'YOUR_API_KEY';
const WEBSITE_KEY = 'EXAMPLE_SITE_KEY';
const WEBSITE_URL = 'https://lessons.zennolab.com/captchas/recaptcha/v3.php?level=beta';
async function solveRecaptcha() {
const browser = await launch({ headless: false });
const page = await browser.newPage();
console.log('Browser is initialized!');
// Initializing the CapMonster client
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));
try {
await page.goto(WEBSITE_URL, {
waitUntil: 'domcontentloaded'
});
console.log('Browser page is switched to captcha page!');
const recaptchaV3ProxylessRequest = new RecaptchaV3ProxylessRequest({
websiteURL: WEBSITE_URL,
websiteKey: WEBSITE_KEY,
minScore: 0.7,
pageAction: "verify",
});
// Getting a captcha solution from CapMonster
const solution = await cmcClient.Solve(recaptchaV3ProxylessRequest);
console.log('CapMonster Solution:', solution);
await page.setUserAgent('Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/126.0.0.0 Safari/537.36');
await page.evaluate((result) => {
document.querySelector('#g-recaptcha-response-100000').style.display = 'block';
document.querySelector('#g-recaptcha-response-100000').style.visibility = 'visible';
document.querySelector('#g-recaptcha-response-100000').value = result;
}, solution);
console.log('Result embedded into the page!');
await Promise.all([
page.waitForNavigation(),
page.click('#v3_submit')
]);
console.log('Button "Check" clicked!');
await new Promise(resolve => setTimeout(resolve, 10000));
} catch (e) {
console.error('An error occurred:', e);
} finally {
await browser.close();
}
}
solveRecaptcha();
- Let's run the code:
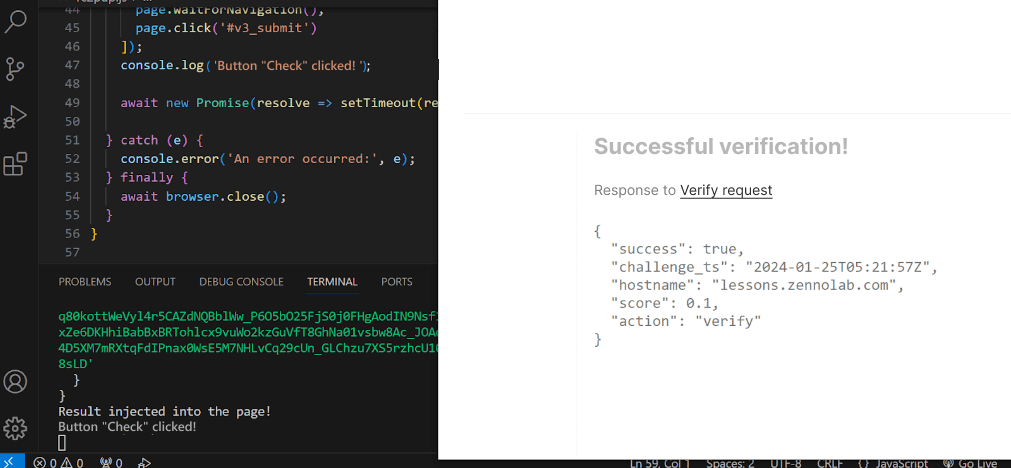
Cloudflare Turnstile
Here we will fill out a small contact form on the test site tsmanaged.zlsupport.com and solve a Cloudflare Turnstile captcha. As for previous types of captchas, we need to find out the sitekey of the captcha in the developer tools in the browser.
- Let's import the dependencies and write the values:
import { launch } from 'puppeteer';
import { CapMonsterCloudClientFactory, ClientOptions, TurnstileProxylessRequest } from '@zennolab_com/capmonstercloud-client';
const CAPMONSTER_API_KEY = 'YOUR_API_KEY';
const WEBSITE_KEY = 'EXAMPLE_SITE_KEY';
const WEBSITE_URL = 'www.example.com';
- Let's launch the browser, create a task for CapMonster Cloud, send it to the server, receive and insert the result into the page:
async function solveCloudflareTurnstileCaptcha() {
const browser = await launch({ headless: false });
const page = await browser.newPage();
// Initializing the CapMonster client
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));
try {
await page.goto(WEBSITE_URL);
console.log('Page is open, solving Cloudflare Turnstile CAPTCHA...');
await page.type('#username', 'login');
await page.type('#password', 'pass');
const turnstileTaskRequest = new TurnstileProxylessRequest({
websiteURL: WEBSITE_URL,
websiteKey: WEBSITE_KEY,
});
// Getting a captcha solution from CapMonster
const solutionObject = await cmcClient.Solve(turnstileTaskRequest);
console.log('CapMonster Solution:', solutionObject);
const token = solutionObject.solution.token;
console.log('Captcha solved:', token);
// Inserting a token into the "token" field
await page.evaluate((extractedToken) => {
const tokenField = document.querySelector('#token');
if (tokenField) {
tokenField.value = extractedToken;
}
}, token);
console.log('Token inserted into the "token" field!');
// Click on the "Submit" button after inserting the token
await page.evaluate(() => {
const submitButton = document.querySelector('button[type="submit"]');
if (submitButton) {
submitButton.click();
}
});
console.log('Clicked on the "Submit" button after token insertion!');
await new Promise(resolve => setTimeout(resolve, 5000));
} catch (error) {
console.error('Error:', error);
} finally {
await browser.close();
}
}
solveCloudflareTurnstileCaptcha();
Explanation of this part of the code:
async function solveCloudflareTurnstileCaptcha() { ... }: Declaring an asynchronous function solveCloudflareTurnstileCaptcha, which contains all the code to solve the Cloudflare Turnstile CAPTCHA.
const browser = await launch({ headless: false });: Launching a browser using Puppeteer. The headless: false option specifies that the browser will be launched with a GUI.
const page = await browser.newPage();: Creating a new tab (page) in the browser.
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));: Initializing the CapMonster client using the provided API key.
await page.goto(WEBSITE_URL);: Going to page with Cloudflare Turnstile CAPTCHA.
console.log('Page opened, solving Cloudflare Turnstile CAPTCHA...');: Displaying a message to the console indicating that the page is open and the process of solving the Cloudflare Turnstile CAPTCHA has begun.
await page.type('#username', 'login');: Data entry in the "username" field.
await page.type('#password', 'pass');: Data entry in the "password" field.
const turnstileTaskRequest = new TurnstileProxylessRequest({ ... });:Creating a request object for the Turnstile CAPTCHA solution. Set parameters such as website URL and Turnstile CAPTCHA key.
const solutionObject = await cmcClient.Solve(turnstileTaskRequest);: Sending a request to CapMonster for the Turnstile CAPTCHA solution. The result is saved in a solutionObject.
console.log('CapMonster Solution:', solutionObject);: Displaying information about the solution from CapMonster to the console.
const token = solutionObject.solution.token;: Retrieving a token (solution result) from a solutionObject.
console.log('Captcha solved:', token);: Displaying a message to the console that the CAPTCHA has been solved and displaying the token.
await page.evaluate((extractedToken) => { ... }, token);: Inserting a token into the "token" field on a web page using Puppeteer's evaluate method.
console.log('Token inserted into the "token" field!');: Displaying a message to the console indicating that a token has been inserted into the "token" field.
await page.evaluate(() => { ... });: Click on the "Submit" button after inserting the token using the Puppeteer evaluate method.
console.log('Clicked on the "Submit" button after token insertion!');: Output a message to the console indicating that a click operation was performed on the "Submit" button after inserting a token.
await new Promise(resolve => setTimeout(resolve, 5000));: Pause for 5 seconds using Promise.
} catch (error) { console.error('Error:', error); } finally { await browser.close(); }:Handling errors (if they occur) with error message output to the console. Closing the browser in a finally block to ensure completion.
solveCloudflareTurnstileCaptcha();: Calling the solveCloudflareTurnstileCaptcha function to complete the entire process of solving the Cloudflare Turnstile CAPTCHA.
Full code:
import { launch } from 'puppeteer';
import { CapMonsterCloudClientFactory, ClientOptions, TurnstileProxylessRequest } from '@zennolab_com/capmonstercloud-client';
const CAPMONSTER_API_KEY = 'YOUR_API_KEY';
const WEBSITE_KEY = 'EXAMPLE_SITE_KEY';
const WEBSITE_URL = 'www.example.com';
async function solveCloudflareTurnstileCaptcha() {
const browser = await launch({ headless: false });
const page = await browser.newPage();
// Initializing the CapMonster client
const cmcClient = CapMonsterCloudClientFactory.Create(new ClientOptions({ clientKey: CAPMONSTER_API_KEY }));
try {
await page.goto(WEBSITE_URL);
console.log('Page opened, solving Cloudflare Turnstile CAPTCHA...');
await page.type('#username', 'login');
await page.type('#password', 'pass');
const turnstileTaskRequest = new TurnstileProxylessRequest({
websiteURL: WEBSITE_URL,
websiteKey: WEBSITE_KEY,
});
// Getting a captcha solution from CapMonster
const solutionObject = await cmcClient.Solve(turnstileTaskRequest);
console.log('CapMonster Solution:', solutionObject);
const token = solutionObject.solution.token;
console.log('Captcha solved:', token);
// Inserting a token into the "token" field
await page.evaluate((extractedToken) => {
const tokenField = document.querySelector('#token');
if (tokenField) {
tokenField.value = extractedToken;
}
}, token);
console.log('Token inserted into the "token" field!');
// Click on the "Submit" button after inserting the token
await page.evaluate(() => {
const submitButton = document.querySelector('button[type="submit"]');
if (submitButton) {
submitButton.click();
}
});
console.log('Clicked on the "Submit" button after token insertion!');
await new Promise(resolve => setTimeout(resolve, 5000));
} catch (error) {
console.error('Error:', error);
} finally {
await browser.close();
}
}
solveCloudflareTurnstileCaptcha();
- Let's run the code. If everything is done correctly, the code will solve the captcha and open a confirmation page:
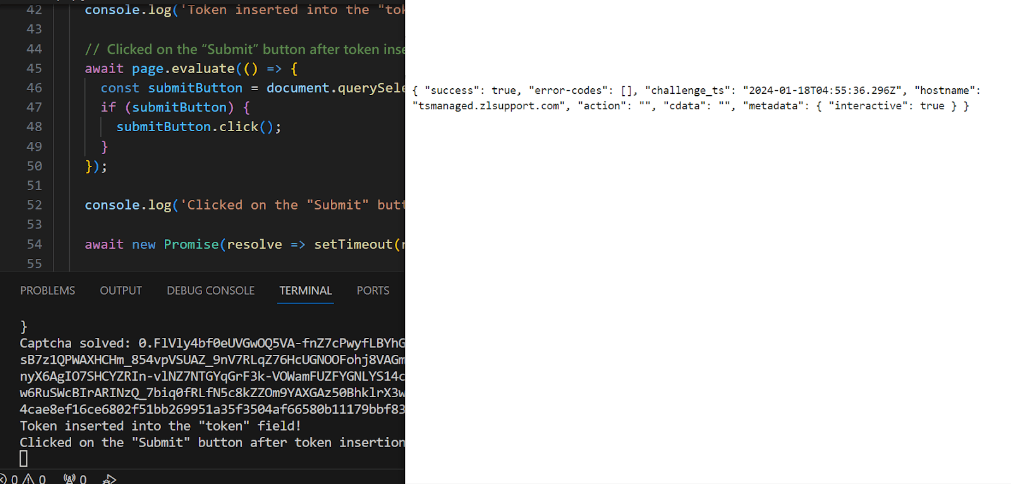
Playwright
Previously we used Puppeteer in JavaScript. Now, let's try using Playwright in Python. We will continue to do the work in VS Code, here are the steps to install Playwright:
- Installing Playwright. Create a new folder where the project files will be located, open it in VS Code, run the following command in your terminal: pip install playwright
- Installing browsers. After installing Playwright, to install all supported browsers (Chromium, Firefox, WebKit) at once run the command: python -m playwright install
To install only one browser, for example Chromium, run the command: python -m playwright install chromium
Install the official CapMonster Cloud library with the command pip install capmonstercloudclient.
Now you can write code to automatically solve captcha.
ReCaptcha v.2
- Import all the necessary dependencies and write down the data (you can create a separate.env file for the data):
import asyncio
from playwright.async_api import async_playwright
from capmonstercloudclient import CapMonsterClient, ClientOptions
from capmonstercloudclient.requests import RecaptchaV2ProxylessRequest
API_KEY = "YOUR_API_KEY"
WEBSITE_URL = "https://lessons.zennolab.com/captchas/recaptcha/v2_simple.php?level=high"
WEBSITE_KEY = "EXAMPLE_SITE_KEY"
- Create a task to send it to the server, using the available data, we will get the answer and insert the solution into the captcha form:
client_options = ClientOptions(api_key=API_KEY)
cap_monster_client = CapMonsterClient(options=client_options)
async def solve_captcha():
# Creating a reCAPTCHA solution request type V2 Proxyless
recaptcha_request = RecaptchaV2ProxylessRequest(websiteUrl=WEBSITE_URL, websiteKey=WEBSITE_KEY)
return await cap_monster_client.solve_captcha(recaptcha_request)
async def main():
try:
async with async_playwright() as p:
# Launching the browser
browser = await p.chromium.launch(headless=False)
context = await browser.new_context()
page = await context.new_page()
# Opening a website
await page.goto(WEBSITE_URL)
print('Page is open, waiting for reCAPTCHA to load...')
# Wait a while for the CAPTCHA to load (you can configure the duration)
await asyncio.sleep(5)
# reCAPTCHA solution using CapMonster
responses = await solve_captcha()
print('Ответ от CapMonster:', responses)
# Inserting a solved reCAPTCHA into a page
await page.evaluate(f'document.getElementById("g-recaptcha-response").innerHTML = "{responses["gRecaptchaResponse"]}";')
print('The result is inserted into the page!')
# Performing additional actions if necessary (for example, submitting a form)
await page.click('#recaptcha-demo-submit')
print('Form is submitted!')
# Wait a while to observe changes
await asyncio.sleep(10)
except Exception as e:
print('Error:', e)
finally:
# Closing the browser
await browser.close()
asyncio.run(main())
Explanation of this part of the code:
client_options = ClientOptions(api_key=API_KEY): Initializing CapMonster client settings using the provided API key.
cap_monster_client = CapMonsterClient(options=client_options): Creating a CapMonster client using the options defined in client_options.
async def solve_captcha(): ...: Asynchronous function solve_captcha, which creates a captcha solution request of type reCAPTCHA V2 Proxyless and passes it to the CapMonster client for solving.
async def main(): ...: Asynchronous main function that includes the main code to open the browser, load the page, solve the captcha using CapMonster, insert the solution into the page, and perform additional actions.
async with async_playwright() as p: ...: Using async_playwright to control the browser with Playwright asynchronously.
browser = await p.chromium.launch(headless=False): Launching the Chromium browser with the ability to display a graphical interface (not in headless mode).
await page.goto(WEBSITE_URL): Going to the specified website.
await asyncio.sleep(5): Pause to wait for CAPTCHA to load (in this case, 5 seconds).
responses = await solve_captcha(): Calling solve_captcha function to solve reCAPTCHA using CapMonster.
await page.evaluate(f'document.getElementById("g-recaptcha-response").innerHTML = "{responses["gRecaptchaResponse"]}";'): Inserting a reCAPTCHA solution into a page using the Playwright evaluate method.
await page.click('#recaptcha-demo-submit'): Click on a button with the specified selector (in this case, the "Submit" button on the reCAPTCHA demo page).
await asyncio.sleep(10): Pause to observe changes after submitting the form (in this case, 10 seconds).
await browser.close(): Closing the browser in a finally block to complete.
asyncio.run(main()): Running the asynchronous main function.
Full code:
import asyncio
from playwright.async_api import async_playwright
from capmonstercloudclient import CapMonsterClient, ClientOptions
from capmonstercloudclient.requests import RecaptchaV2ProxylessRequest
API_KEY = "YOUR_API_KEY"
WEBSITE_URL = "https://lessons.zennolab.com/captchas/recaptcha/v2_simple.php?level=high"
WEBSITE_KEY = "EXAMPLE_SITE_KEY"
client_options = ClientOptions(api_key=API_KEY)
cap_monster_client = CapMonsterClient(options=client_options)
async def solve_captcha():
# Creating a reCAPTCHA solution request type V2 Proxyless
recaptcha_request = RecaptchaV2ProxylessRequest(websiteUrl=WEBSITE_URL, websiteKey=WEBSITE_KEY)
return await cap_monster_client.solve_captcha(recaptcha_request)
async def main():
try:
async with async_playwright() as p:
# Launching the browser
browser = await p.chromium.launch(headless=False)
context = await browser.new_context()
page = await context.new_page()
# Opening a website
await page.goto(WEBSITE_URL)
print('Page is open, waiting for reCAPTCHA to load...')
# Wait a while for the CAPTCHA to load (you can configure the duration)
await asyncio.sleep(5)
# reCAPTCHA solution using CapMonster
responses = await solve_captcha()
print('CapMonster response:', responses)
# Inserting a solved reCAPTCHA into a page
await page.evaluate(f'document.getElementById("g-recaptcha-response").innerHTML = "{responses["gRecaptchaResponse"]}";')
print('The result is inserted into the page!')
# Perform additional actions if necessary (for example, submitting a form)
await page.click('#recaptcha-demo-submit')
print('Form is submitted!')
# Wait a while to observe changes
await asyncio.sleep(10)
except Exception as e:
print('Error:', e)
finally:
# Closing the browser
await browser.close()
asyncio.run(main())
Successful captcha solution:
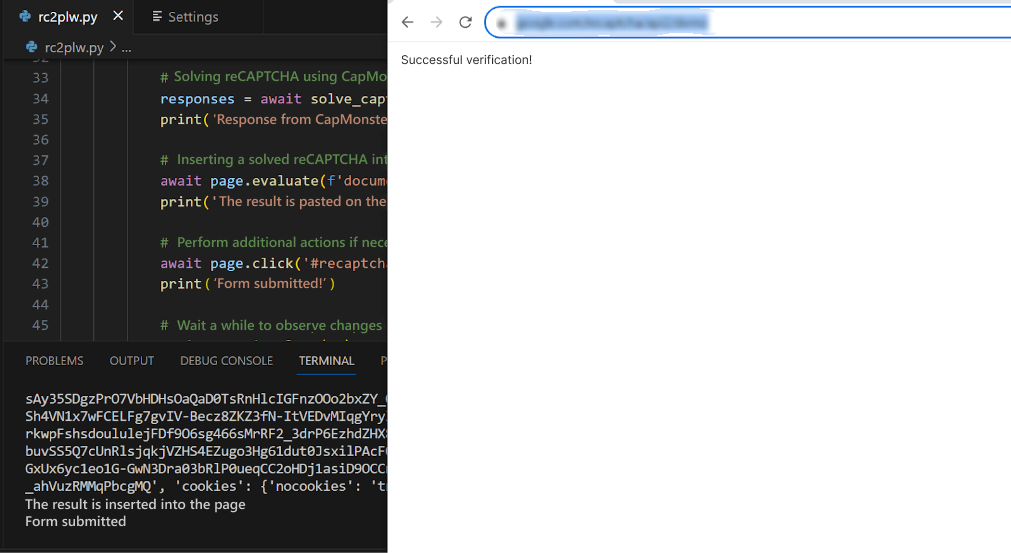
ReCaptcha v.3
- Import all the necessary dependencies and enter the data:
import asyncio
from playwright.async_api import async_playwright
from capmonstercloudclient import CapMonsterClient, ClientOptions
from capmonstercloudclient.requests import RecaptchaV3ProxylessRequest
CAPMONSTER_API_KEY = 'YOUR_API_KEY'
WEBSITE_KEY = 'EXAMPLE_SITE_KEY'
WEBSITE_URL = 'https://lessons.zennolab.com/captchas/recaptcha/v3.php?level=beta'
- Create a task, send it to the server and get a solution:
async def solve_recaptcha():
# Initializing Playwright
async with async_playwright() as p:
browser = await p.chromium.launch(headless=False)
context = await browser.new_context()
page = await context.new_page()
print('Browser initialized!')
# Initializing the CapMonster client
client_options = ClientOptions(api_key=CAPMONSTER_API_KEY)
cap_monster_client = CapMonsterClient(options=client_options)
try:
# Setting a custom userAgent
(optional)
user_agent = 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/126.0.0.0 Safari/537.36' #Insert yours
await page.set_extra_http_headers({'User-Agent': user_agent})
# Going to the specified page
await page.goto(WEBSITE_URL, wait_until='domcontentloaded')
print('Browser switched to page with captcha!')
# Creating a request for a reCAPTCHA solving type V3 Proxyless
recaptcha_request = RecaptchaV3ProxylessRequest(
websiteUrl=WEBSITE_URL,
websiteKey=WEBSITE_KEY,
action='verify',
min_score=0.7,
)
# reCAPTCHA solving using CapMonster
task_result = await cap_monster_client.solve_captcha(recaptcha_request)
print('reCAPTCHA V3 solved:', task_result)
# Inserting the result into the page
await page.evaluate(f'document.querySelector(\'#g-recaptcha-response-100000\').style.display = "block";')
await page.evaluate(f'document.querySelector(\'#g-recaptcha-response-100000\').style.visibility = "visible";')
await page.evaluate(f'document.querySelector(\'#g-recaptcha-response-100000\').value = "{task_result}";')
print('The result is inserted into the page!')
# Click on the button to check
await page.click('#v3_submit')
print('"Check" button pressed!')
# Pause for 10 seconds
await page.wait_for_timeout(10000)
finally:
# Closing the browser
await browser.close()
# Running an asynchronous function to solve reCAPTCHA
asyncio.run(solve_recaptcha())
Explanation of this part of the code:
async with async_playwright() as p: ...: Using async_playwright to control the browser with Playwright asynchronously.
browser = await p.chromium.launch(headless=False): Launching the Chromium browser with the ability to display a graphical interface (not in headless mode).
await page.goto(URL): Going to the specified website.
client_options = ClientOptions(api_key=API_KEY): Initializing CapMonster client settings using the provided API key.
cap_monster_client = CapMonsterClient(options=client_options): Creating a CapMonster client using the options defined in client_options.
recaptcha_request = RecaptchaV3ProxylessRequest(...: Creating a request object for the reCAPTCHA V3 Proxyless solution. Here are set parameters such as website URL, reCAPTCHA key, action and minimum score.
task_result = await cap_monster_client.solve_captcha(recaptcha_request): reCAPTCHA solving using CapMonster. The result is saved in task_result.
await page.evaluate(f'document.querySelector(\'#g-recaptcha-response-100000\').style.display = "block";'): Inserting the result into the page using the Playwright evaluate method. In this case, the style of the element is changed so that it becomes visible.
await page.click('[data-action="demo_action"]'): Click on the button with the specified selector (in this case, the “Check” button).
await asyncio.sleep(10): Pause for 10 seconds to observe changes.
await browser.close(): Closing the browser in a finally block to complete.
asyncio.run(solve_recaptcha()): Running the asynchronous solve_recaptcha function.
Full code:
import asyncio
from playwright.async_api import async_playwright
from capmonstercloudclient import CapMonsterClient, ClientOptions
from capmonstercloudclient.requests import RecaptchaV3ProxylessRequest
CAPMONSTER_API_KEY = 'YOUR_API_KEY'
WEBSITE_KEY = 'EXAMPLE_SITE_KEY'
WEBSITE_URL = 'https://lessons.zennolab.com/captchas/recaptcha/v3.php?level=beta'
async def solve_recaptcha():
# Initializing Playwright
async with async_playwright() as p:
browser = await p.chromium.launch(headless=False)
context = await browser.new_context()
page = await context.new_page()
print('Browser initialized!')
# Initializing the CapMonster client
client_options = ClientOptions(api_key=CAPMONSTER_API_KEY)
cap_monster_client = CapMonsterClient(options=client_options)
try:
# Installing the user agent
user_agent = 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/126.0.0.0 Safari/537.36'
await page.set_extra_http_headers({'User-Agent': user_agent})
# Going to the specified page
await page.goto(WEBSITE_URL, wait_until='domcontentloaded')
print('Browser switched to page with captcha!')
# Creating a request for a reCAPTCHA solution type V3 Proxyless
recaptcha_request = RecaptchaV3ProxylessRequest(
websiteUrl=WEBSITE_URL,
websiteKey=WEBSITE_KEY,
action='verify',
min_score=0.7,
)
# reCAPTCHA solution using CapMonster
task_result = await cap_monster_client.solve_captcha(recaptcha_request)
print('reCAPTCHA V3 solved:', task_result)
# Inserting the result into the page
await page.evaluate(f'document.querySelector(\'#g-recaptcha-response-100000\').style.display = "block";')
await page.evaluate(f'document.querySelector(\'#g-recaptcha-response-100000\').style.visibility = "visible";')
await page.evaluate(f'document.querySelector(\'#g-recaptcha-response-100000\').value = "{task_result}";')
print('The result is inserted into the page!')
# Click on the button to check
await page.click('#v3_submit')
print('"Check" button pressed!')
# Pause for 10 seconds
await page.wait_for_timeout(10000)
finally:
# Closing the browser
await browser.close()
# Running an asynchronous function to solve reCAPTCHA
asyncio.run(solve_recaptcha())
- If everything is done correctly, the code will automatically solve the captcha and open a page with successful verification:
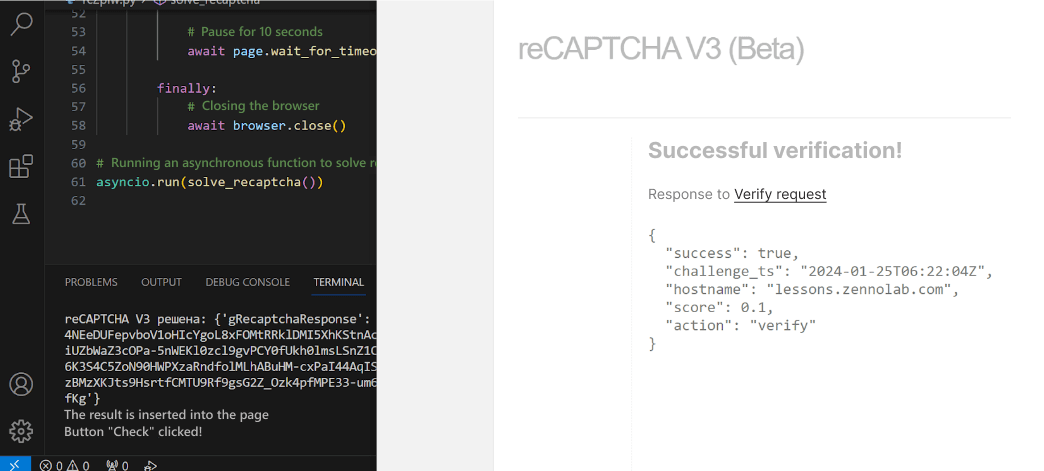
Cloudflare Turnstile
- Import the required dependencies:
import asyncio
from playwright.async_api import async_playwright
from capmonstercloudclient import CapMonsterClient, ClientOptions
from capmonstercloudclient.requests import TurnstileProxylessRequest
- Enter the data (or import it from a .env file):
API_KEY = 'YOUR_API_KEY'
WEBSITE_KEY = 'EXAMPLE_SITE_KEY'
WEBSITE_URL = 'www.example.com'
- Create a task, send it to the CapMonster Cloud server to solve the captcha, get the result and insert the token into the appropriate captcha element:
async def solve_turnstile_captcha():
async with async_playwright() as p:
browser = await p.chromium.launch(headless=False)
context = await browser.new_context()
page = await context.new_page()
try:
await page.goto(WEBSITE_URL)
print('Page opened, solving Cloudflare Turnstile CAPTCHA...')
# Fill in the "username" and "password" fields with your credentials
await page.fill('input[name="username"]', 'yourlogin')
await page.fill('input[name="password"]', 'yourpass')
# Initializing the CapMonster client
client_options = ClientOptions(api_key=API_KEY)
cap_monster_client = CapMonsterClient(options=client_options)
turnstile_request = TurnstileProxylessRequest(
websiteURL=WEBSITE_URL,
websiteKey=WEBSITE_KEY,
)
# Cloudflare Turnstile CAPTCHA Solution Using CapMonster
task_result = await cap_monster_client.solve_captcha(turnstile_request)
token = task_result.get('token')
print('Captcha solved:', token)
# Inserting a token into the "token" field
await page.evaluate(f'document.querySelector(\'#token\').value = \'{token}\';')
print('Token inserted into the "token" field!')
# Clicking the "Submit" button after inserting the token
await page.click('button[type="submit"]')
print('Clicked on the "Submit" button after token insertion!')
await asyncio.sleep(5)
except Exception as e:
print(f"An error occurred: {e}")
finally:
await browser.close()
asyncio.run(solve_turnstile_captcha())
Explanation of this part of the code:
async with async_playwright() as p: ...: Using async_playwright to control the browser with Playwright asynchronously.
browser = await p.chromium.launch(headless=False):Launching the Chromium browser with the ability to display a graphical interface (not in headless mode).
context = await browser.new_context():Creating a new context for a page.
page = await context.new_page(): Creating a new page in a new context.
await page.goto(WEBSITE_URL): Going to the specified website.
await page.fill('input[name="username"]', 'yourlogin'): Filling the "username" field with the specified login.
await page.fill('input[name="password"]', 'yourpass'): Filling the "password" field with the specified password.
client_options = ClientOptions(api_key=API_KEY): Initializing CapMonster client settings using the provided API key.
cap_monster_client = CapMonsterClient(options=client_options): Creating a CapMonster client using the options defined in client_options.
turnstile_request = TurnstileProxylessRequest(...: Creating a request object for the Cloudflare Turnstile CAPTCHA Proxyless solution. Here are set parameters such as website URL and key.
task_result = await cap_monster_client.solve_captcha(turnstile_request): Cloudflare Turnstile CAPTCHA solution using CapMonster. The result is saved in task_result.
token = task_result.get('token'): Retrieving a token from solution results.
await page.evaluate(f'document.querySelector(\'#token\').value = \'{token}\';'): Inserting a token into the "token" field using Playwright's evaluate method.
await page.click('button[type="submit"]'): Clicking the "Submit" button.
await asyncio.sleep(5): Pause for 5 seconds to observe changes.
await browser.close(): Closing the browser in a finally block to complete.
asyncio.run(solve_cloudflare_turnstile_captcha()): Running the asynchronous function solve_cloudflare_turnstile_captcha.
Full code:
import asyncio
from playwright.async_api import async_playwright
from capmonstercloudclient import CapMonsterClient, ClientOptions
from capmonstercloudclient.requests import TurnstileProxylessRequest
API_KEY = 'YOUR_API_KEY'
WEBSITE_KEY = 'EXAMPLE_SITE_KEY'
WEBSITE_URL = 'www.example.com'
async def solve_turnstile_captcha():
async with async_playwright() as p:
browser = await p.chromium.launch(headless=False)
context = await browser.new_context()
page = await context.new_page()
try:
await page.goto(WEBSITE_URL)
print('Page opened, solving Cloudflare Turnstile CAPTCHA...')
# Fill in the "username" and "password" fields with your data
await page.fill('input[name="username"]', 'yourlogin')
await page.fill('input[name="password"]', 'yourpass')
# Initializing the CapMonster client
client_options = ClientOptions(api_key=API_KEY)
cap_monster_client = CapMonsterClient(options=client_options)
turnstile_request = TurnstileProxylessRequest(
websiteURL=WEBSITE_URL,
websiteKey=WEBSITE_KEY,
)
# Cloudflare Turnstile CAPTCHA solution using CapMonster
task_result = await cap_monster_client.solve_captcha(turnstile_request)
token = task_result.get('token')
print('Captcha solved:', token)
# Inserting a token into the "token" field
await page.evaluate(f'document.querySelector(\'#token\').value = \'{token}\';')
print('Token inserted into the "token" field!')
# Clicking the "Submit" button after inserting the token
await page.click('button[type="submit"]')
print('Clicked on the "Submit" button after token insertion!')
await asyncio.sleep(5)
except Exception as e:
print(f"An error occurred: {e}")
finally:
await browser.close()
asyncio.run(solve_turnstile_captcha())
- Run the project and see the result. If everything is done correctly, you’ll see the page with the completed verification:
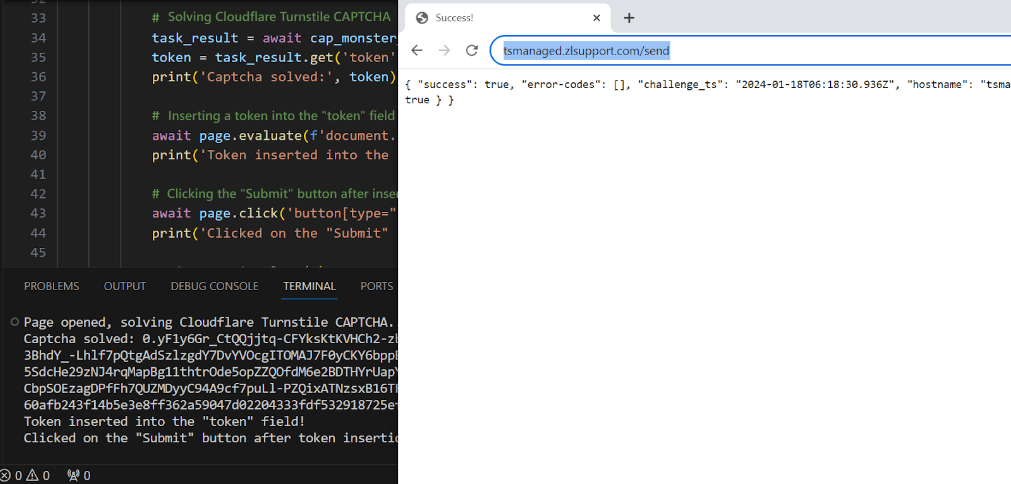
Conclusion
As you can see, bypassing captchas only seems like a difficult task. All you need is to know the site data and the captcha data, to indicate its type and correctly create a task, send it and receive/insert the token into the required element. CapMonster Cloud will do the rest. It can be used together with almost any libraries and frameworks, and integrating this service with Puppeteer or Playwright using code will allow you to quickly and efficiently get solutions to even the most complex captchas on the necessary sites.
Note: We'd like to remind you that the product is used for automating testing on your own websites and on websites to which you have legal access.